Payments - Embedded
A simple guide to using embedded payments on your website
Here is how to accept embedded card payments. The API reference can be found here
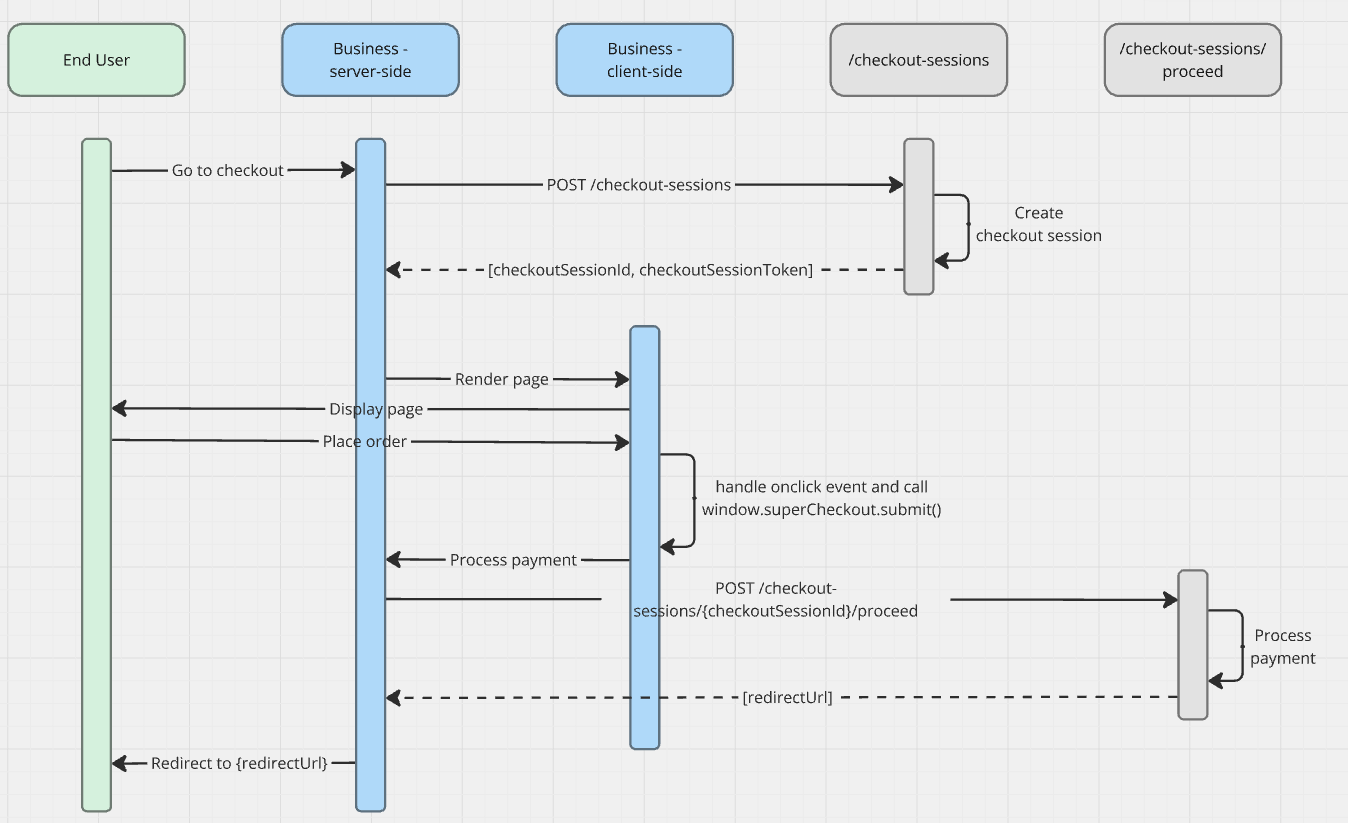
Create a checkout Session
Before you render your checkout page, make a server side call to create a checkout session. A checkout session is necessary to initiate and manage the payment process.
This call returns a checkoutSessionToken
, which is needed in subsequent steps.
Note: Make sure this API call is made on the server side to keep your API key secure.
curl --request POST \
--url https://api.superpayments.com/2024-10-01/checkout-sessions \
--header 'Authorization: <<apiKey>>'
Render your checkout page
When you render your checkout page, make sure you have included payment.js
and added the <super-checkout>
web component. The component will handle taking the payment.
This component requires two properties:
checkout-session-token
: This property should be set to the token you received from the server side call.amount
: This property represents the current cart value. Make sure to keep it updated to reflect any changes in the cart amount.
<html>
<head>...</head>
<body>
...
<script src="https://cdn.superpayments.com/js/payment.js"></script>
<super-checkout amount="CART_AMOUNT" checkout-session-token="CHECKOUT_SESSION_TOKEN_FROM_STEP_1"></super-checkout>
...
</body>
</html>
Customise the look and feel to match the design of your site
Super Payments Checkout supports visual customisation, which allows you to match the design of your site.
theme
property with all the supported styles
const theme = JSON.stringify({
hideSuperApp: true, // 'true' to hide "Pay with the Super app"
tabStyles: {
backgroundColor: 'transparent',
selectedBackgroundColor: '#F7F6F4',
borderColor: '#c8a865',
borderRadius: '0px',
borderWidth: '2px',
color: '#848EA6',
},
container: {
backgroundColor: '#F7F6F4',
borderRadius: '0px',
},
title: { // only applies when `title` property is being used
fontFamily: 'Tagesschrift',
fontSize: '30px',
color: '#848EA6',
},
});
<super-checkout amount='CART_AMOUNT' checkout-session-token='CHECKOUT_SESSION_TOKEN' theme='THEME_OBJECT'></super-checkout>
Note: the theme object needs to be stringify because of how WebComponent works
title
andsubtitle
properties will allow to change the message to the users and will remove the merchant logo.
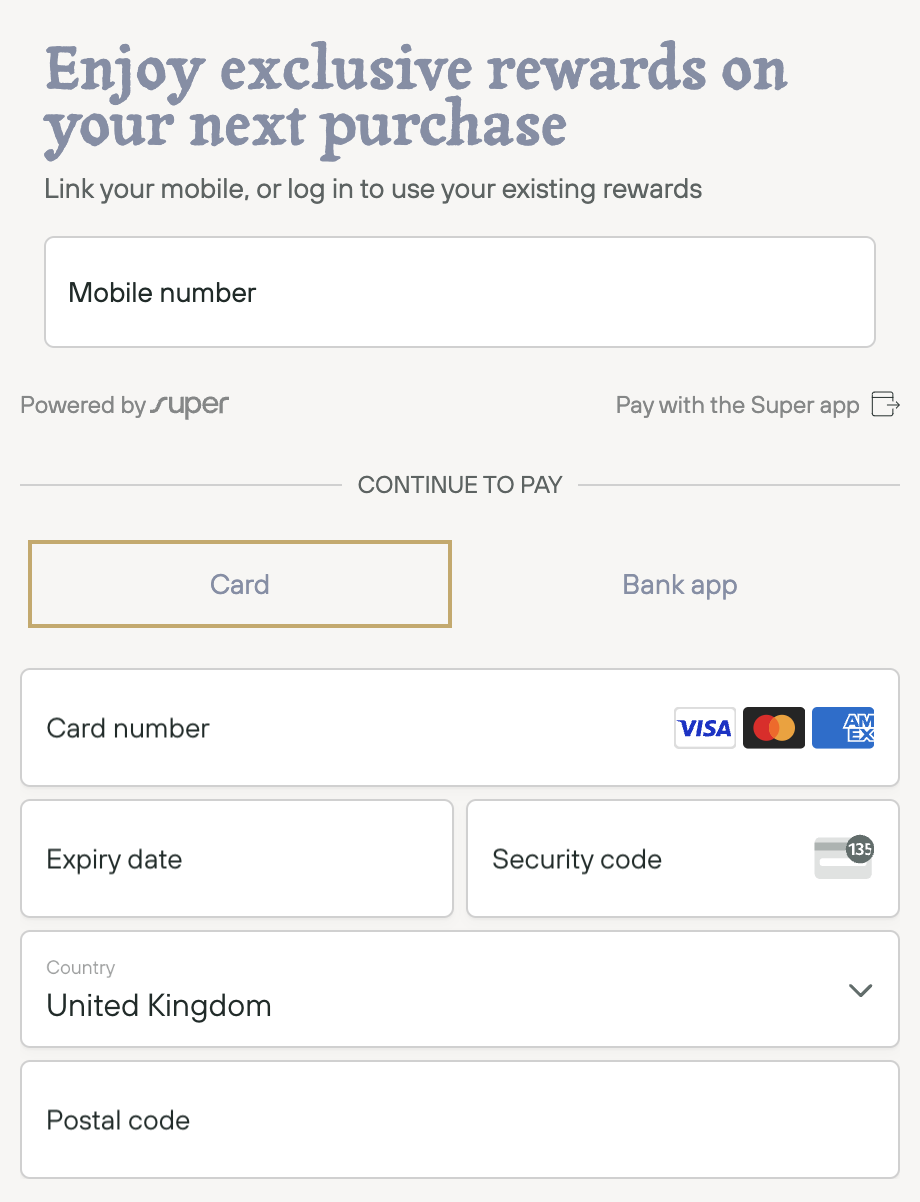
Example using the "theme", "title" and "subtitle" properties
Initiate a payment
When the you are ready to take a payment, call window.superCheckout.submit()
to allow the embedded <super-checkout>
component to complete its necessary steps before proceeding with the payment process.
This can be done by intercepting the click event on your "Place Order" button
<button onclick="handlePlaceOrderClicked()">Place Order</button>
<script>
async function handlePlaceOrderClicked() {
const result = await window.superCheckout.submit();
// Handle any failures appropriately for your checkout, for example...
if (result.status === "FAILURE") {
if (result.errorMessage) {
throw new Error(result.errorMessage);
} else {
// Include a default error message in case nothing was received on the failure response.
throw new Error("Something went wrong. No money has been taken from your account. Please refresh the page and try again.");
}
} else if (result.status === "SUCCESS") {
// Continue to server-side processing
}
}
</script>
Success Result
If the checkout is successful, you will receive the following:
{
"status": "SUCCESS"
}
Continue to server-side processing to complete the payment
Failure Result
If the checkout fails, you will receive the following:
{
"status": "FAILURE",
"errorMessage": "Your card has insufficient funds."
}
Prevent server-side processing and display the error message. If no specific error message is included, display a generic message like:
"Something went wrong. No money has been taken from your account. Please refresh the page and try again."
Complete the payment
After receiving a success result from window.superCheckout.submit()
, make a server-side call to the proceed
endpoint to continue the checkout session. This ensures that the payment process proceeds securely on the server.
Note: This API call should be made on the server side to keep your API key secure.
curl --request POST \
--url https://api.superpayments.com/2024-10-01/checkout-sessions/{checkoutSessionId}/proceed \
--header 'Authorization: <<apiKey>>' \
--header 'Content-Type: application/json' \
-d '{
"amount": CART_AMOUNT,
"cancelUrl": "https://your-site.com/cancel",
"failureUrl": "https://your-site.com/failure",
"successUrl": "https://your-site.com/success",
"externalReference": "your_external_reference",
"email": "[email protected]",
"phone": "1234567890"
}'
After the proceed call, redirect the user to the redirectUrl
provided in the response. This will either:
- Redirect the customer to one of the
successUrl
,failureUrl
, orcancelUrl
you specified earlier. - Redirect the customer to Super Payments to complete further actions, such as opening their bank app for authentication.
In the case of additional steps required by the customer (e.g., authentication via bank app), the redirectUrl will guide them through the next steps before finalizing the payment.
Handle Post-Payment Events
Set up a webhook to handle post-payment events sent by Super. These events notify your system of payment status updates even if the customer closes the browser or leaves the page before the callback completes.
Listening to these asynchronous events ensures a more robust integration and support for various payment methods.
Tip: For more details on setting up webhooks, check out our webhook documentation.
Updated 7 days ago