Super provides a suite of components you can embed directly in your website by using our embedded javascript.
When the script is included, a superjs
object is placed in the global namespace. The SDK must be initialized, once during its lifecycle, by calling the function superjs.init()
with the required arguments.
<body>
<!-- other site content goes here -->
<script src="https://cdn.superpayments.com/js/super.js"></script>
<script>
superjs.init(<YOUR_PUBLISHABLE_KEY>, { integrationId: <YOUR_INTEGRATION_ID>, platform: <YOUR_PLATFORM>, page: <YOUR_PAGE> });
</script>
</body>
When including the JS SDK via a <script>
tag it should be loaded synchronously. It's recommended to place the <script>
tag at the bottom of the <body>
element to avoid blocking the document from rendering.
Obtaining your publishable key
We will shortly make the
PUBLISHABLE_KEY
andINTEGRATION_ID
available in the business portal, but in the mean time you can make a request directly to the api using yourSUPER_API_KEY
to get the values:curl https://api.superpayments.com/business-config -H "Authorization: $SUPER_API_KEY" { "currentIntegration": "xxxxxxxxx", "publishableKeys": [ { "key": "PUB_XXXXXXXX" } ] }
Init Options
The superjs.init()
function has two required arguments. The first argument is the Publishable Key. This is a unique key associated with a business and is used to authenticate with Super's backend services. Unlike the Private API Key, the Publishable Key has very limited capabilities and is suitable for embedding in a public web page.
The second function argument includes options for configuring the SDK. The following table describes these attributes, including any that are mandatory.
Option | Description | Type | Mandatory? | Default? |
---|---|---|---|---|
integrationId | ID used to determine which integration to use. | string | Yes | N/A |
page | The page the SDK is being initialised on. | 'home' , 'cart' , 'checkout' , 'product-listing' , 'product-detail' | Yes | N/A |
platform | The e-commerce platform being used (if any). | 'woo-commerce' , 'shopify' , 'magento' , 'big-commerce' , 'custom' | Yes | N/A |
platformVersion | The version number of the e-commerce platform. | string | No | "unknown" |
superPluginVersion | The version of the e-commerce Super plugin (if any). | string | No | "unknown" |
sdkVersion | The Super JS SDK version. This is set automatically. | string | No | "unknown" |
The mandatory SDK options, integrationId
, page
, and platform
, are used to determine the display theme and layout of the Web Components on a merchant's website. Each merchant has the capability to configure these on a per-page, and per-integration basis. The non-mandatory attributes are primarily used for debugging.
Components
super-banner

It takes the following attributes:
This is the full-width banner component that can be displayed at the top of any page.
Option | Type | Default |
---|---|---|
cartAmount | number | 0 |
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
colorScheme | ColorScheme | "orange" |
Example usage:
Place this where you want the component displayed
<super-banner cartamount="0" page="product-listing" cartid="cart1" colorscheme="orange" cartitems="[]"></super-banner>
super-cart-callout

This is a "callout" element that can be placed on a page to highlight any offers from Super. Clicking on the callout triggers a description modal to open, that further explains & breaks down the particular offer. For this component, the offer is derived from the current cart amount.
It takes the following attributes:
Option | Type | Default |
---|---|---|
cartAmount | number | 0 |
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
placement | main , drawer | main |
position | string | "primary" |
width | string | 'null' |
Example usage:
Place this where you want the component displayed
<super-cart-callout cartamount="1800" page="product-listing" cartid="examplecart" cartItems="[]"></super-cart-callout>
super-product-callout

This is a "callout" element that can be placed on a page to highlight any offers from Super. Clicking on the callout triggers a description modal to open, that further explains & breaks down the particular offer. For this component, the offer is derived from a single product and is suitable for product detail/product listing pages.
It takes the following attributes:
Option | Type | Default |
---|---|---|
productAmount | number | 0 |
productQuantity | number | 1 |
cartId | string | "unknown" |
page | Page | "unknown" |
width | string | null |
Example usage:
Place this where you want the component displayed
<super-product-callout productamount="1800" page="product-listing" cartid="examplecart"></super-product-callout>
super-payment-method-title

This is a dynamic title component that serves as a call-to-action for a user to pay with Super, and includes the expected offer amount that a customer can expect to receive by paying with Super. These calculations are based on the cart total. It is typically used on the checkout page of an e-commerce platform, if the platform allows for dynamic content/HTML to be part of the payment method title.
It takes the following attributes:
Option | Type | Default |
---|---|---|
cartAmount | number | 0 |
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
super-payment-method-description

This is description/explainer component that provides an overview of Super's overall value proposition, and benefit to customers. It includes the expected offer amount that a customer can expect to receive by paying with Super. These calculations are based on the cart total. It is typically used on the checkout page of an e-commerce platform, near the super-payment-method-title
, as a way of enticing a customer to pay with Super.
It takes the following attributes:
Option | Type | Default |
---|---|---|
cartAmount | number | 0 |
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
super-referral-callout
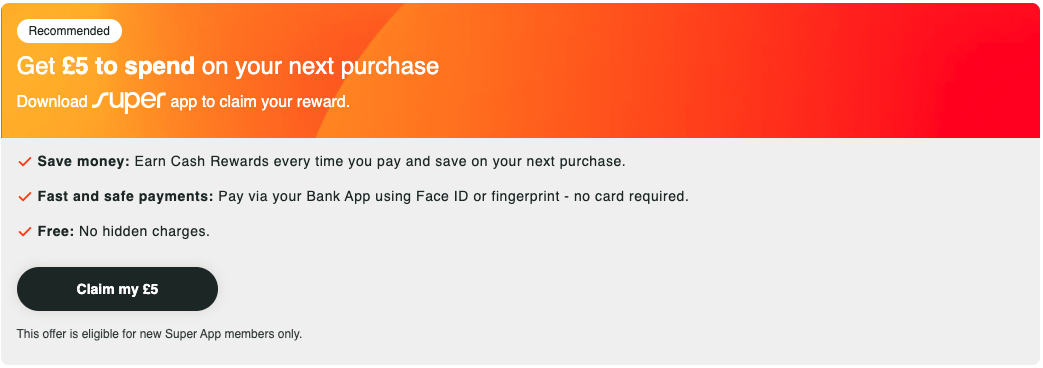
This is a large callout component that can be used to encourage a customer to sign-up for Super via the Refer-a-Customer scheme. It includes a unique referral link that they may use to sign-up, and describes the offer than can expect to receive. It is typically used on the order confirmation page of an e-commerce platform, after a customer has paid with a non-Super payment method.
N.B. The offer described in this component is currently not dynamic, and will always appear as £5.
It currently takes no attributes.
Shared Types
Name | Type |
---|---|
Page | 'home' , 'cart' , 'checkout' , 'product-listing' , 'product-detail' , 'unknown' |
ColorScheme | 'black-orange' , 'black-white' , 'yellow' , 'orange' , 'white-orange' , 'white-black' |
super-checkout-button

This is the checkout button that takes the customer to the Super checkout form. It is customizable from the Admin Portal where we can change its text, color, border, width and logo.
It takes the following attributes:
Option | Type | Default |
---|---|---|
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
amount | number | 0 |
`productQuantity' | number | 1 |
width | string | " " |
fontStyles | string | " " |
position | string | "primary" |
super-checkout-form
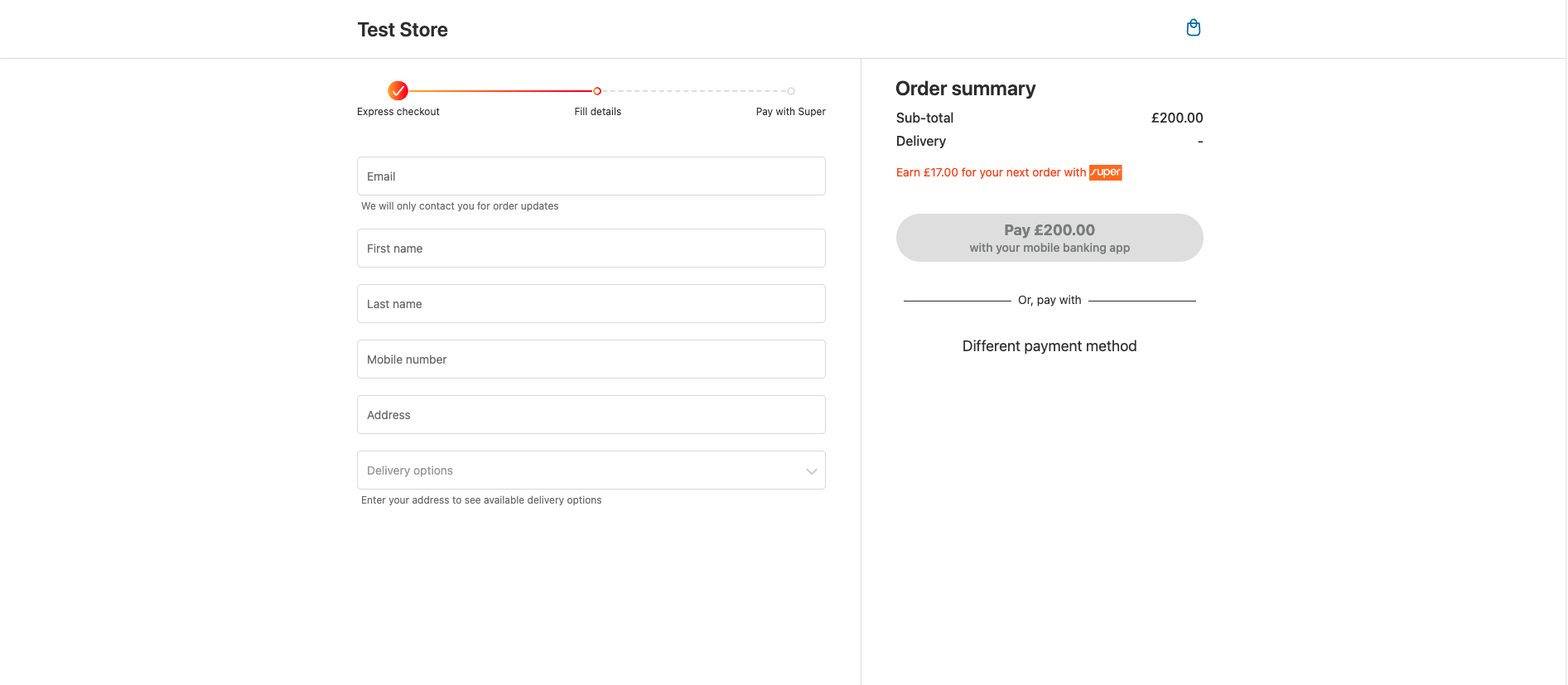
This is the Super checkout form where the customer can fill in its details like: name, email, address, delivery method and proceed with the payment. It is customizable from the Admin Portal where we can change the fields, panel color, font color etc.
It takes the following attributes:
Option | Type | Default |
---|---|---|
storeName | string | `` |
cartId | string | "unknown" |
cartItems | CartItem[] | [] |
page | Page | "unknown" |
cartAmount | number | 0 |
storeLink | string | "/" |
cartLink | string | "/cart" |
countries | CountryCode[] | ["GB"] |
storeId | string | unknown |